Firebase Cloud Messaging
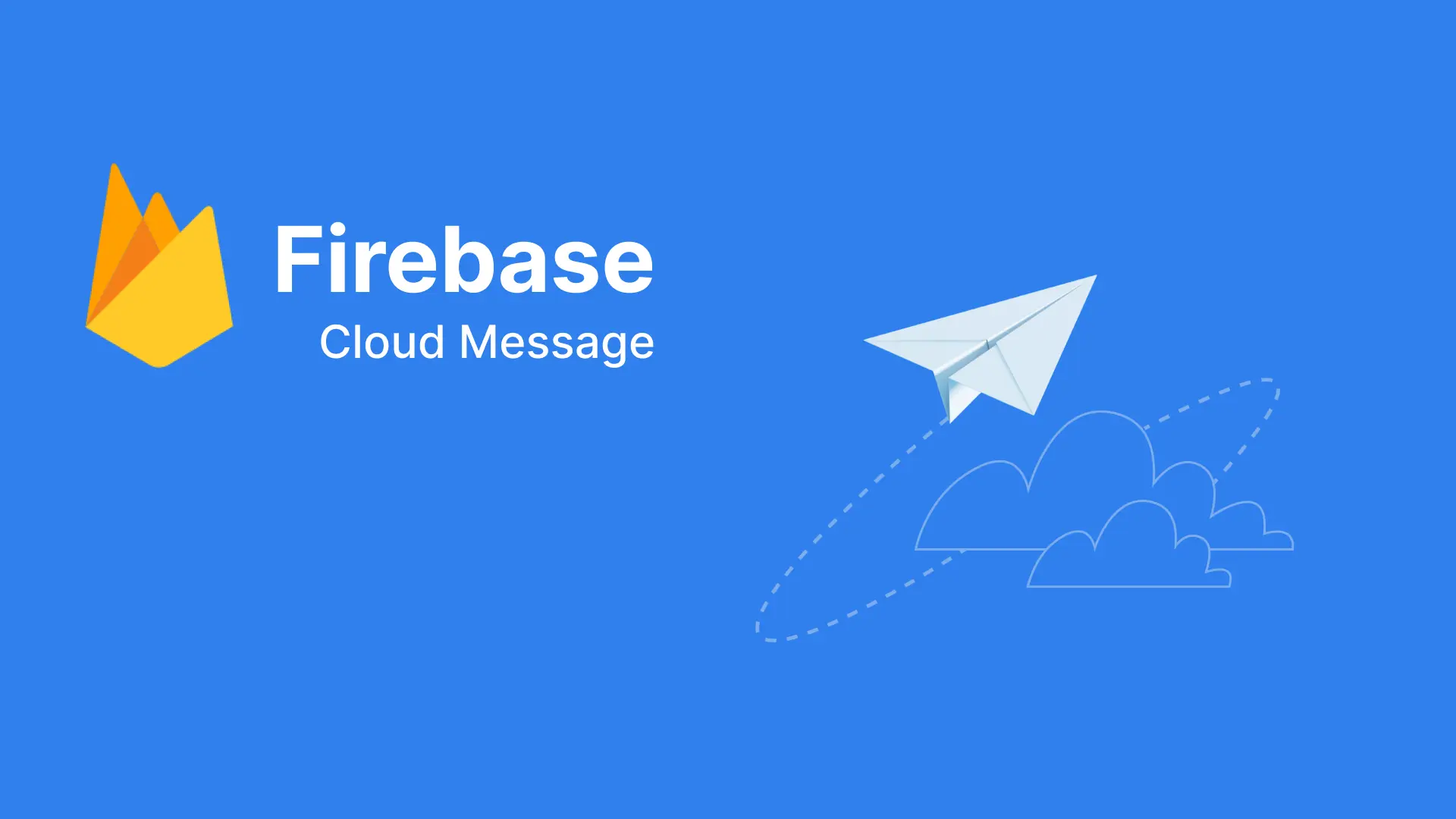
I. FCM Architecture
Firebase Cloud Messaging (FCM) is a service provided by Google as part of Firebase to facilitate the sending of notifications and messages from the server to mobile devices, web applications, and other devices.
FCM architecture:
How FCM works
Registering the device:
- When users install an application with the Firebase SDK on their mobile device or website, the device will register with FCM.
- Firebase will generate a unique identification token for this device.
Send token to server:
- The token is sent from the device to the application's server, where it is stored for future use.
Send notifications from the server:
- When the server application wants to send a notification to registered devices, it uses FCM's API to send the message along with a list of target device tokens.
FCM sends notifications to the device:
- FCM will receive the message from the server and deliver it to all registered devices.
Message processing on device:
- The device that receives a notification from FCM displays it to the user.
FCM applications are not just limited to sending notifications, but can also be integrated to perform tasks such as data synchronization, user authentication, and many other features to improve user experience. used on diverse platforms.
Pay attention in managing FCM tokens, it will change in the following cases:
- The app is restored on the new device
- App is removed/reinstalled
- User actively deletes app data
II. Demo with Flutter
Continuing with the demo project from the previous tutorial, let's add the library to the Flutter project:
- flutter pub add firebase_messaging
Requesting permission
Open a notification requesting permission to receive notifications when a user opens the app:
FirebaseMessaging messaging = FirebaseMessaging.instance;
NotificationSettings settings = await messaging.requestPermission(
alert: true,
announcement: false,
badge: true,
carPlay: false,
criticalAlert: false,
provisional: false,
sound: true,
);
print('User granted permission: ${settings.authorizationStatus}');
After the customer grants permission to receive notifications, the application can handle messages sent with cases such as the following:
1.Foreground messages
Forground is the state when the application is open, and the user is actively viewing or using it. To listen to messages while your app is in the foreground, listen for onMessage:
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Got a message whilst in the foreground!');
print('Message data: ${message.data}');
if (message.notification != null) {
print('Message also contained a notification: ${message.notification}');
}
});
Access the console -> Go to Messaging -> Create a test message as follows:
When sent notification to the device, we will receive the corresponding data in the onMessage method.
2.Background messages
This is the state when the application is in the background (when the app is being used, and the user presses the home button or switches to another app). Use onBackgroundMessage to receive messages in this case:
FirebaseMessaging.onBackgroundMessage(FCM.firebaseMessagingBackgroundHandler);
When the app is minimized to the background, and a notification is sent from the console, we receive the notification successfully with the following log:
3. App Killed
When the app is killed, we cannot observe logs like in the two previous cases. Instead, the notification will be displayed directly in the device's notification center as follows:
In the case of not receiving notifications during debugging when the app is killed, you can access and further check according to the instructions:
https://stackoverflow.com/questions/57054706/flutter-fcm-is-not-working-when-app-is-closed
https://github.com/firebase/flutterfire/issues/9685
Note:
For iOS, access Project Settings -> Choose Cloud Messaging -> Upload APNs certificates so that the application can receive notifications. Additionally, notifications are only received on real devices.