Firebase Cloud FireStore
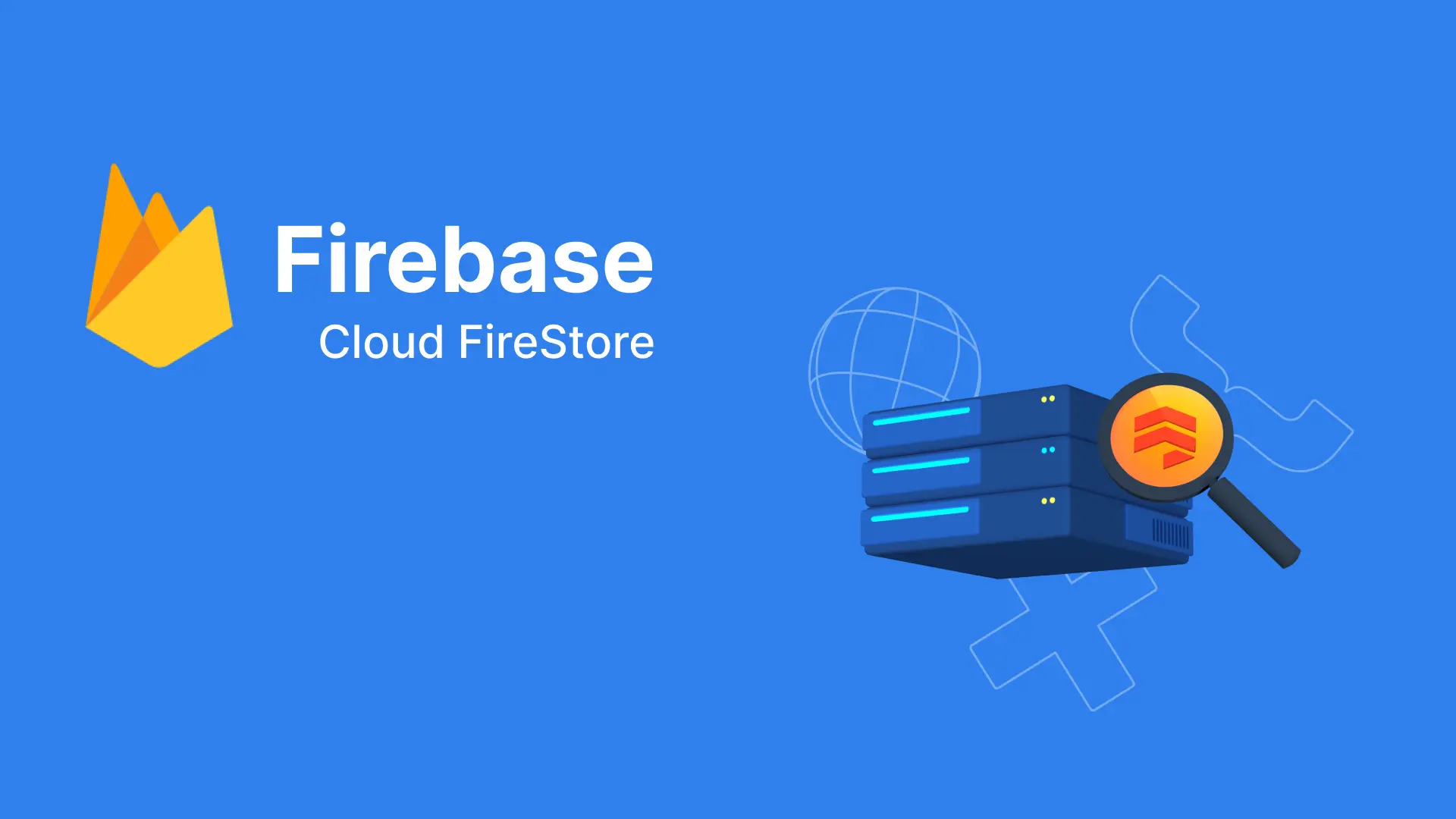
I. Overview
Cloud Firestore is a NoSQL database from Google Firebase, designed to store and synchronize data across mobile, web, and server applications.
Cloud Firestore organizes data as documents within collections. Each document contains fields and values, creating a flexible data structure that reflects the application's architecture:
Collection -> Document -> Fields
One of the notable features of Cloud Firestore is its real-time update capability. Data is automatically synchronized across devices, ensuring that the application always maintains the latest information.
II.Pricing
According to the latest information: https://firebase.google.com/pricing
- The number of documents you read, write, and delete.
- The number of index entries that match composite queries. You are charged once for reading a document for each batch with a maximum of 1000 index entries matching the query.
- The storage capacity your database uses, including fees for metadata and indexing.
- Network bandwidth.
1. Document writes
The cost of writing data in Firebase's Cloud Firestore depends on the number of times you perform write operations, including adding new, updating data.
Document writes are calculated as follows:
Add, set, or update operation is counted as one write.
====== Adding a New Document
var newDocumentRef = FirebaseFirestore.instance.collection('users').add({
'name': 'John Doe',
'age': 25,
});
// Each time you add a new document is considered a write operation.
====== Update a Field in a Document
// Update the 'age' field in a document
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
userDocRef.update({
'age': 26,
});
// Each time you update a field in a document is considered a write operation
===== Batch Writes
// Use batch writes to add and update multiple documents in a batch
var batch = FirebaseFirestore.instance.batch();
// Add new document
batch.set(FirebaseFirestore.instance.collection('users').doc('user1'), {'name': 'Alice', 'age': 30});
// Update document
batch.update(FirebaseFirestore.instance.collection('users').doc('user2'), {'age': 28});
batch.commit();
// The total write count is the sum of the number of times each operation is performed in the batch
===== Atomic Transactions
// Use transactions to perform a write operation atomically
FirebaseFirestore.instance.runTransaction((transaction) async {
// Read the current data
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
var snapshot = await transaction.get(userDocRef);
var currentAge = snapshot.data()?['age'] ?? 0;
// Update data
transaction.update(userDocRef, {'age': currentAge + 1});
});
// Each time a transaction is executed is considered a write operation
2. Document deletes
===== Delete an individual document
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
userDocRef.delete();
// Each time you delete a document is considered a document delete.
===== Batch write with delete operations
// Use batch writes to delete multiple documents in a batch
var batch = FirebaseFirestore.instance.batch();
// Delete document
batch.delete(FirebaseFirestore.instance.collection('users').doc('user1'));
// Delete other document
batch.delete(FirebaseFirestore.instance.collection('users').doc('user2'));
batch.commit();
// The total delete count is the sum of the number of times each delete operation is performed in the batch.
===== Atomic Transactions with Delete Operations
// Use transactions to perform a delete operation atomically
FirebaseFirestore.instance.runTransaction((transaction) async {
// Delete document
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
transaction.delete(userDocRef);
});
// Each time a transaction with a delete operation is executed is considered a document delete.
3. Document reads
Document Reads are calculated based on the number of documents actually read from the database, not the number of documents returned by the query.
===== Read Document
// Read a document from the collection
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
var snapshot = await userDocRef.get();
// Each time you read a document is considered a Document Read
===== Query Multiple Documents
// Execute a query to read multiple documents
var querySnapshot = await FirebaseFirestore.instance.collection('users').where('age', isGreaterThan: 25).get();
//Each time you read a document within the query result is a Document Read. For example, if your query in the database matches 10 documents, then the Document Read count is considered as 10.
Additionally, there is a minimum fee for each document read for each query you perform, even if the query doesn't return any results.
===== Aggregation queries
For aggregation queries such as count(), sum(), and avg(), you are charged one document read for each batch of up to 1000 index entries matched by the query. For aggregation queries that match 0 index entries, there is a minimum charge of one document read.
For example, count() operations that match between 0 and 1000 index entries are billed for one document read. For a count() operation that matches 1500 index entries, you are billed 2 document reads.
===== Read Through Stream
// Read data through a stream with a specific user_id
var userDocRef = FirebaseFirestore.instance.collection('users').doc('user_id');
var stream = userDocRef.snapshots();
// Each time there is a change in the data is considered a Document Read
// Read data from a collection through a stream with an entire collection
var collectionRef = FirebaseFirestore.instance.collection('users');
var stream = collectionRef.snapshots();
// The first time, Firebase will send a snapshot with the entire data in 'users'
// The Document Reads cost will depend on the number of documents in 'users'. For example, if there are 10 users, it will be 10 Document Reads
// After that, whenever there is a change in 'users', Firebase will send snapshots of the updates
// The Document Reads cost for each update will depend on the number of documents changed in that update
III. Demo
Create database
Note: The location, once selected, cannot be changed.
Temporarily set allow read, write to true to allow reading and writing to Firestore.
Add lib:
- flutter pub add cloud_firestore
Add new data and check on the console.
To read data in the collection, we have: