Firebase Authentication
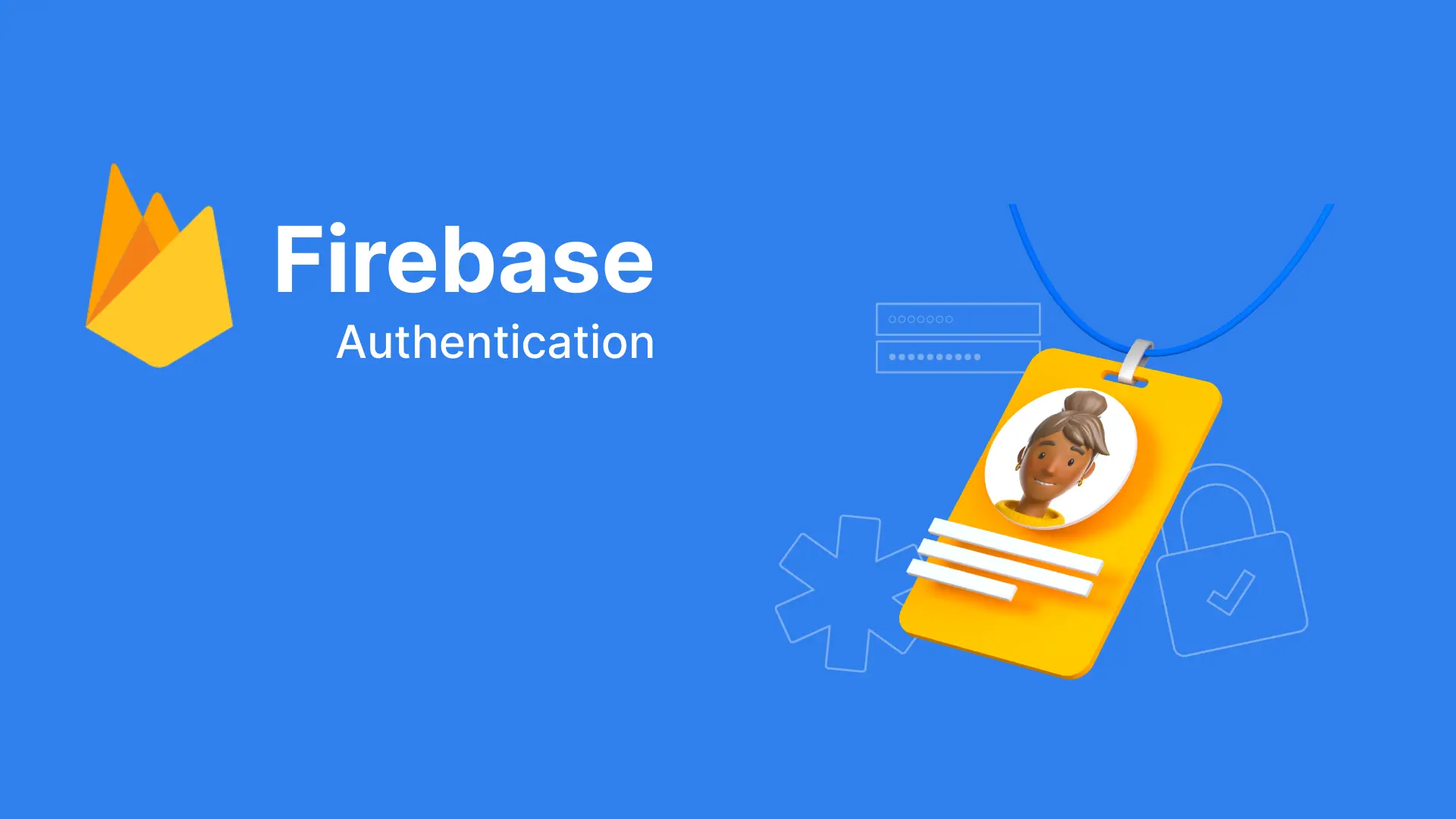
I. Overview
Firebase Authentication operates on a client-server model, where users access and interact with your application from the client side, and the Firebase Authentication service is deployed on the Firebase server. Here is how Firebase Authentication works in detail:
Registration and Login:
- When users want to use your application, they can either register (if they don't have an account) or log in (if they already have an account).
- Allow users to use various login methods such as email/password, phone number, or even login with accounts from Google, Facebook, Twitter, etc.
SDK Integration:
- Your application utilizes the Firebase Authentication SDK to integrate authentication services into the source code.
- Firebase provides SDKs for various platforms such as Android, iOS, and web.
Token-Based Authentication:
- When a user successfully logs in, Firebase Authentication creates a JSON Web Token (JWT) containing user information.
- This JWT is securely sent and stored on the user's device and is used to authenticate access when interacting with Firebase services and resources.
Real-Time Authentication State:
- Firebase provides a mechanism to track the real-time authentication state of users.
- When the authentication state changes (login or logout), this event is emitted, and the application can respond immediately.
Firebase Authentication provides a comprehensive and easy-to-integrate solution for managing user authentication. It not only enhances the security of your application but also improves the user experience by offering convenient and secure login methods.
II. Demo with Flutter
Demo integrating Authentication with email/password
Go to Firebase Authentication -> Sign-in method -> Email/Password -> Enable
Add lib:
- flutter pub add firebase_auth
First, we need to add a user:
After adding a user in Console -> Authentication -> Users, we will see that the user has been successfully added.
The user has been created, now if authentication is required during login, we will use:
Run debug and check the received results: